Keeping your website data fresh and up-to-date can be a challenge, especially when some information resides on external platforms. Manually fetching and integrating data from third-party sources can be time-consuming and error-prone. Fortunately, WordPress offers built-in functionalities like custom Cron jobs that can automate these processes. In this blog post, we’ll delve into integrating third-party APIs with custom WP Cron jobs, allowing you to seamlessly exchange data and keep your website dynamic.
Before diving into the technical aspects, let’s establish the context. Imagine you run a travel blog and want to display real-time currency exchange rates on your website. Manually updating these rates every day wouldn’t be ideal. This is where third-party APIs come in. Many currency exchange services provide APIs that allow you to retrieve up-to-date rates programmatically. By integrating such an API with a custom WP Cron job, you can automate the process of fetching the latest rates and displaying them on your website, ensuring your visitors always have access to accurate information.
The benefits of integrating APIs with custom Cron jobs are numerous. Firstly, it automates data retrieval, freeing you from manual updates. Secondly, it guarantees data accuracy by pulling information directly from the source. Finally, it improves website functionality by seamlessly integrating external data, enhancing the user experience.
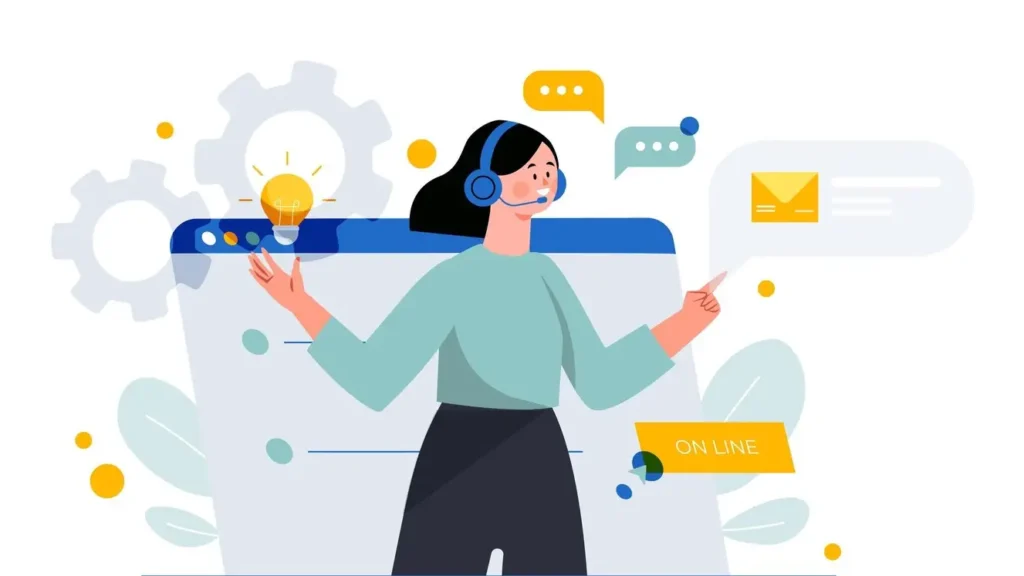
Now, let’s get down to business! Planning your integration is crucial for a smooth execution. First, identify the specific API you want to connect with. Many popular services offer well-documented APIs, making integration easier. Next, determine the specific data points you need. In our currency exchange example, it would be the exchange rates for specific currencies. Additionally, decide on the update frequency. Do you need hourly updates for real-time accuracy, or are daily updates sufficient? Finally, consider security. Most APIs require authentication, so obtain the necessary API keys or credentials securely and store them appropriately (we’ll discuss security best practices later).
With the planning phase complete, we can move on to the implementation. We’ll break it down into manageable steps, including code snippets for each stage.
1. Setting Up Custom Cron Job
The first step involves scheduling a custom Cron job to call our API function at the desired interval. Here’s where WP Cron jobs come into play. These built-in functions allow you to execute code periodically at specific times or intervals. Here’s a code snippet demonstrating how to schedule a custom Cron job using the wp_schedule_event
function:
PHP
// Define the hook name and interval
$hook_name = 'my_currency_update';
$interval = 'hourly'; // Adjust interval as needed (e.g., 'daily')
// Check if the Cron job is already scheduled
if ( ! wp_next_scheduled( $hook_name ) ) {
// Schedule the Cron job
wp_schedule_event( time(), $interval, $hook_name );
}
// Define the function to be executed by the Cron job
add_action( $hook_name, 'my_currency_update_function' );
This code snippet first defines the hook name (my_currency_update
) and the desired interval (hourly
). It then checks if a Cron job with that name already exists. If not, it schedules the Cron job using wp_schedule_event
, specifying the current time, the chosen interval, and the hook name. Finally, it associates the my_currency_update_function
with the my_currency_update
hook, ensuring the function is called when the Cron job runs.
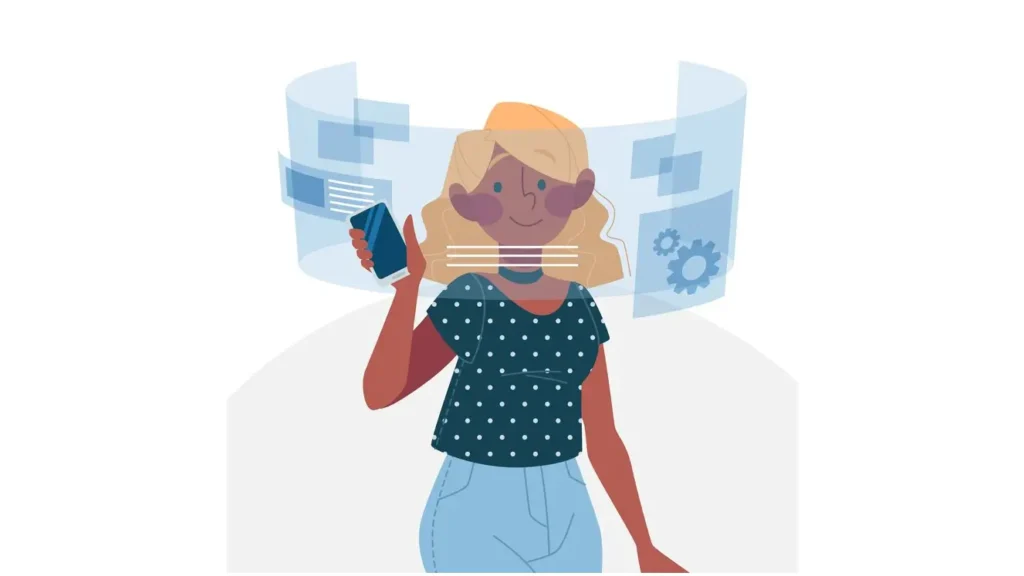
2. Making the API Call
Within the my_currency_update_function
, we’ll make the actual API call to retrieve the exchange rates. WordPress offers various functionalities for making HTTP requests. Here, we’ll use the wp_remote_get
function to fetch data from the API endpoint.
Here’s a code snippet demonstrating the API call within the function:
PHP
function my_currency_update_function() {
// Define the API endpoint URL
$api_url = 'https://api.example.com/v1/rates';
// Build the request arguments (if necessary)
$args = array(
'headers' => array(
'Authorization' => 'Bearer YOUR_API_KEY', // Replace with your actual API key
),
);
// Make the API call using wp_remote_get
$response = wp_remote_get( $api_url, $args );
// Process the API response (see next section)
}
This code snippet defines the API endpoint URL. If your API requires authentication, you’ll need to include the necessary headers
in the $args
array, replacing YOUR_API_KEY
with your actual API key. Finally, it uses wp_remote_get
to make the API call, storing the response in the $response
variable.
3. Processing the API Response
The next step involves processing the response received from the API. The format of the response will depend on the specific API you’re using, but it’s commonly JSON. We’ll need to decode the response and extract the relevant data. Here’s a code snippet demonstrating how to handle the response:
PHP
function my_currency_update_function() {
// ... (code for making the API call)
if ( is_wp_error( $response ) ) {
// Handle potential errors (see next section)
return;
}
$data = json_decode( wp_remote_retrieve_body( $response ) );
if ( ! is_object( $data ) || ! isset( $data->rates ) ) {
// Handle invalid response format
return;
}
// Extract the exchange rates from the response
$exchange_rates = $data->rates;
// Utilize the fetched data (see next section)
}
This code snippet first checks if the $response
is a WordPress error object. If it is, we’ll need to handle the error gracefully (discussed later). Assuming a successful response, it uses wp_remote_retrieve_body
to get the response body and then decodes the JSON data using json_decode
. It then performs some basic validation to ensure the response format is as expected. Finally, it extracts the relevant data (exchange rates in this case) from the decoded object and stores it in the $exchange_rates
variable.
4. Utilizing the Fetched Data
Now that we have the retrieved data, we can use it for various purposes. Here are a couple of examples:
- Updating Posts or Pages: If you have a dedicated page displaying currency exchange rates, you can update the content with the fetched data. This could involve using the WordPress API to update specific post fields or directly modifying the page content using custom code.
- Creating Dynamic Content: You can leverage the exchange rates to create dynamic content on your website. For instance, you could display a currency conversion calculator or integrate the rates into product pricing if your website sells products in different currencies.
The specific implementation will depend on your desired functionality. However, here’s a general code snippet demonstrating how you could update a post with the retrieved exchange rates:
PHP
function my_currency_update_function() {
// ... (code for processing the API response)
$post_id = 123; // Replace with the actual post ID for your currency exchange page
$content = 'Current Exchange Rates:';
foreach ( $exchange_rates as $currency => $rate ) {
$content .= "<br> - $currency: $rate";
}
// Update the post content using the WordPress API (or custom code)
wp_update_post( array( 'ID' => $post_id, 'post_content' => $content ) );
}
This code snippet iterates through the retrieved exchange rates and builds a formatted string containing the current rates. Finally, it updates the content of the post with the ID 123
(replace with your actual post ID) using the wp_update_post
function from the WordPress API.
5. Best Practices and Considerations
While integrating APIs with Cron jobs offers numerous benefits, there are some best practices and considerations to keep in mind:
- Rate Limits: Many APIs have rate limits restricting the number of requests you can make within a specific timeframe. Be mindful of these limits and adjust your Cron job interval accordingly.
- Error Handling: Always implement robust error handling to gracefully manage situations where the API call fails or returns unexpected data. Log errors for debugging purposes and consider notifying an administrator if critical errors occur.
- Security: As mentioned earlier, secure storage and handling of API keys are crucial. Avoid storing them directly in your code. Consider using a WordPress secrets management plugin for secure key storage and retrieval.
- Data Validation: Before utilizing the retrieved data, validate its format and content to ensure it’s what you expect. This helps prevent unexpected behavior on your website.
- Performance Optimization: While custom Cron jobs offer flexibility, excessive API calls can impact website performance. Optimize your code and API requests to minimize server load.
Conclusion
Integrating third-party APIs with custom WP Cron jobs unlocks a world of possibilities for keeping your website dynamic and data-driven. By following the outlined steps and best practices, you can automate data exchange, improve website functionality, and enhance the user experience. Remember to choose well-documented APIs, plan your integration carefully, and implement robust error handling and security measures. By leveraging this powerful technique, you can ensure your website stays up-to-date and delivers valuable information to your visitors.
In Action: A Real-World Example
At OmegaAdvertising, we’ve had the pleasure of working with a high-end salon on their website. We implemented the exact functionality described in this blog post, allowing them to automatically display real-time currency exchange rates on their website, catering to their international clientele. This not only enhances the user experience but also showcases their commitment to providing a seamless and modern salon experience.
Ready to Automate Your Website?
If you’re looking to integrate third-party APIs or automate tasks on your website, we can help! Contact OmegaAdvertising today for a free consultation and discuss how we can streamline your website operations and enhance its functionality.
Here are some additional resources that you might find helpful:
- WordPress Cron Jobs Documentation: https://codex.wordpress.org/Category:WP-Cron_Functions
- wp_remote_get Function Documentation: https://developer.wordpress.org/reference/functions/wp_remote_get/
- Best Practices for WordPress Security: https://www.wpbeginner.com/wordpress-security/
Leave a Reply